24. Swap Nodes in Pairs
Medium
Given a linked list, swap every two adjacent nodes and return its head.
You may not modify the values in the list's nodes. Only nodes itself may be changed.
Example 1:
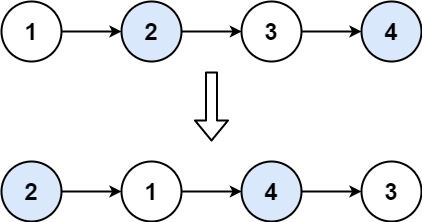
Input: head = [1,2,3,4] Output: [2,1,4,3]
Example 2:
Input: head = [] Output: []
Example 3:
Input: head = [1] Output: [1]
Constraints:
- The number of nodes in the list is in the range
[0, 100]
. 0 <= Node.val <= 100
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | /** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode() {} * ListNode(int val) { this.val = val; } * ListNode(int val, ListNode next) { this.val = val; this.next = next; } * } */ class Solution { public ListNode swapPairs(ListNode head) { if(head==null || head.next==null) return head; ListNode H = new ListNode(); H.next = head; ListNode prev = H, p1 = head, p2 = head.next; while(p2!=null){ ListNode next = p2.next; p2.next = p1; p1.next = next; prev.next = p2; prev = p1; if(next==null || next.next==null) break; p1 = next; p2 = p1.next; } return H.next; } } |
No comments:
Post a Comment