Thursday, February 26, 2015
LeetCode [93] Restore IP Addresses
LeetCode [89] Gray Code
LeetCode [79] Word Search
79. Word Search
Medium
Given a 2D board and a word, find if the word exists in the grid.
The word can be constructed from letters of sequentially adjacent cells, where "adjacent" cells are horizontally or vertically neighboring. The same letter cell may not be used more than once.
Example 1:
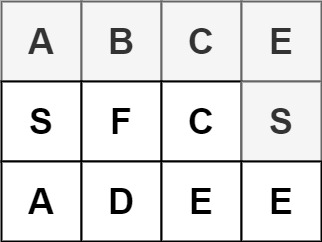
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCCED" Output: true
Example 2:
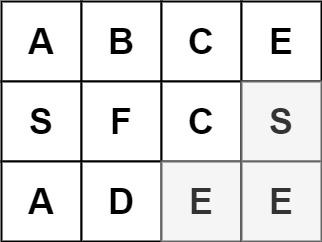
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "SEE" Output: true
Example 3:
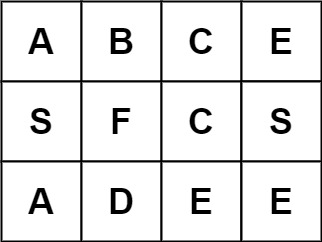
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCB" Output: false
Constraints:
board
andword
consists only of lowercase and uppercase English letters.1 <= board.length <= 200
1 <= board[i].length <= 200
1 <= word.length <= 10^3
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 | class Solution { public: bool bt(vector<vector<char> > &board, string word, bool **passed, int i, int j, int m, int n, int len, int nw){ if(len==nw) return true; //up if(i-1>=0 && board[i-1][j]==word[len] && !passed[i-1][j]){ passed[i-1][j] = true; if(bt(board, word, passed, i-1, j, m, n, len+1, nw)) return true; passed[i-1][j] = false; } //low if(i+1<m && board[i+1][j]==word[len] && !passed[i+1][j]){ passed[i+1][j] = true; if(bt(board, word, passed, i+1, j, m, n, len+1, nw)) return true; passed[i+1][j] = false; } //left if(j-1>=0 && board[i][j-1]==word[len] && !passed[i][j-1]){ passed[i][j-1] = true; if(bt(board, word, passed, i, j-1, m, n, len+1, nw)) return true; passed[i][j-1] = false; } //left if(j+1<n && board[i][j+1]==word[len] && !passed[i][j+1]){ passed[i][j+1] = true; if(bt(board, word, passed, i, j+1, m, n, len+1, nw)) return true; passed[i][j+1] = false; } return false; } bool exist(vector<vector<char> > &board, string word) { int m = board.size(); if(m==0) return false; int n = board[0].size(); int nw = word.size(); if(n==0||nw==0) return false; bool **passed = new bool *[m]; for(int i=0; i<m; ++i){ passed[i] = new bool[n]; } for(int i=0; i<m; ++i){ for(int j=0; j<n; ++j){ if(board[i][j]==word[0]&&!passed[i][j]){ passed[i][j] = true; if(bt(board, word, passed, i, j, m, n, 1, nw)) return true; passed[i][j] = false; } } } return false; } }; class Solution { public: bool exist(vector<vector<char>>& board, string word) { if(word.empty()) return true; int m = board.size(); if(m==0) return false; int n = board[0].size(); if(n==0) return false; string cur; int sz = word.size(); for(int i=0; i<m; ++i){ for(int j=0; j<n; ++j){ char c = word[0]; if(board[i][j]==c){ cur += c; board[i][j] = '.'; if(bt(board, cur, word, 1, i, j, sz, m, n)) return true; board[i][j] = c; } } } return false; } bool bt(vector<vector<char>>& board, string cur, string word, int len, int i, int j, int sz, int m, int n){ if(len==sz) return true; char c = word[len]; string cur1 = cur; cur1 += c; if(i-1>=0 && board[i-1][j]==c){ board[i-1][j] = '.'; if(bt(board, cur1, word, len+1, i-1, j, sz, m, n)) return true; board[i-1][j] = c; } if(i+1<m && board[i+1][j]==c){ board[i+1][j] = '.'; if(bt(board, cur1, word, len+1, i+1, j, sz, m, n)) return true; board[i+1][j] = c; } if(j-1>=0 && board[i][j-1]==c){ board[i][j-1] = '.'; if(bt(board, cur1, word, len+1, i, j-1, sz, m, n)) return true; board[i][j-1] = c; } if(j+1<n && board[i][j+1]==c){ board[i][j+1] = '.'; if(bt(board, cur1, word, len+1, i, j+1, sz, m, n)) return true; board[i][j+1] = c; } return false; } }; class Solution { public: bool exist(vector<vector<char>>& board, string word) { int m = board.size(); if(m==0) return false; int n = board[0].size(); for(int i=0; i<m; ++i){ for(int j=0; j<n; ++j){ if(dfs(board, word, 0, word.size(), i, j, m, n)) return true; } } return false; } bool dfs(vector<vector<char>>& board, string word, int pos, int sz, int i, int j, int m, int n){ if(pos<sz && board[i][j]==word[pos]){ if(pos+1==sz) return true; board[i][j]='#'; if(i-1>=0) if(dfs(board, word, pos+1, sz, i-1, j, m, n)) return true; if(i+1<m) if(dfs(board, word, pos+1, sz, i+1, j, m, n)) return true; if(j-1>=0) if(dfs(board, word, pos+1, sz, i, j-1, m, n)) return true; if(j+1<n) if(dfs(board, word, pos+1, sz, i, j+1, m, n)) return true; board[i][j]=word[pos]; } return false; } }; |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | class Solution { int m, n; int[][] dirs = {{-1,0},{1,0},{0,-1},{0,1}}; public boolean exist(char[][] board, String word) { m = board.length; n = board[0].length; for(int i=0; i<m; ++i){ for(int j=0; j<n; ++j){ if(board[i][j]==word.charAt(0)){ board[i][j] = '.'; if(find(board, word, 1, i, j)) return true; board[i][j] = word.charAt(0); } } } return false; } boolean find(char[][] board, String word, int p, int i, int j){ if(p==word.length()) return true; for(int[] d : dirs){ int ii = i+d[0], jj = j+d[1]; if(ii>=0 && ii<m && jj>=0 && jj<n && board[ii][jj]==word.charAt(p)){ board[ii][jj] = '.'; if(find(board, word, p+1, ii, jj)) return true; board[ii][jj] = word.charAt(p); } } return false; } } |
LeetCode [77] Combinations
77. Combinations
Medium
Given two integers n and k, return all possible combinations of k numbers out of 1 ... n.
You may return the answer in any order.
Example 1:
Input: n = 4, k = 2 Output: [ [2,4], [3,4], [2,3], [1,2], [1,3], [1,4], ]
Example 2:
Input: n = 1, k = 1 Output: [[1]]
Constraints:
1 <= n <= 20
1 <= k <= n
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | //C++ class Solution { public: vector<vector<int>> combine(int n, int k) { vector<vector<int>> res; if(!n||!k) return res; vector<int> cur; bt(n, k, res, cur, 0, 0); } void bt(int n, int k, vector<vector<int>> &res, vector<int> cur, int pos, int size){ if(size==k){ res.push_back(cur); }else if(pos<=n-k+size){ bt(n, k, res, cur, pos+1, size); cur.push_back(pos+1); bt(n, k, res, cur, pos+1, size+1); } } }; //Java class Solution { List<List<Integer>> ret = new ArrayList<>(); public List<List<Integer>> combine(int n, int k) { helper(new ArrayList<>(), n, k, 1); return ret; } void helper(List<Integer> cur, int n, int k, int i) { if(cur.size()==k){ ret.add(new ArrayList<Integer>(cur)); }else{ for(int j=i; j<=n; ++j){ if(n-j+1+cur.size()<k) break; cur.add(j); helper(cur, n, k, j+1); cur.remove(cur.size()-1); } } } } |
LeetCode [52] N-Queens II
LeetCode [51] N-Queens
Wednesday, February 25, 2015
LeetCode [47] Permutations II
47. Permutations II
Medium
Given a collection of numbers, nums
, that might contain duplicates, return all possible unique permutations in any order.
Example 1:
Input: nums = [1,1,2] Output: [[1,1,2], [1,2,1], [2,1,1]]
Example 2:
Input: nums = [1,2,3] Output: [[1,2,3],[1,3,2],[2,1,3],[2,3,1],[3,1,2],[3,2,1]]
Constraints:
1 <= nums.length <= 8
-10 <= nums[i] <= 10
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | class Solution { public: vector<vector<int>> permuteUnique(vector<int>& nums) { vector<vector<int>> ret; sort(nums.begin(), nums.end()); bt(nums, ret, 0, nums.size()); return ret; } //nums cannot be reference so that nums[pos+1..n-1] is in increasing order void bt(vector<int> nums, vector<vector<int>> &ret, int pos, int n){ if(pos==n){ ret.push_back(nums); }else{ for(int i=pos; i<n; ++i){ if(i>pos && nums[i]==nums[pos]) continue; swap(nums[pos], nums[i]); bt(nums, ret, pos+1, n); //cannot swap back; otherwise nums[pos+1..n-1] will not be in increasing order } } } }; |
===========
NoteIt is important to keep the increasing order of the non-determined portion of the vector, ie., nums[pos+1, n-1], such that we can conveniently skip the duplicate cases by line 17.
An example for the recursion of nums. pos=0. Note that nums[1, 4] are in increasing order.
0 1 2 3 4 -- index
1 2 3 4 5
2 1 3 4 5
3 1 2 4 5
4 1 2 3 5
5 1 2 3 4
If nums is swapped back at line20. nums[1, 4] are no longer in increasing order.
0 1 2 3 4 -- index
1 2 3 4 5
2 1 3 4 5
3 2 1 4 5
4 2 3 1 5
5 2 3 4 1
Labels:
Backtracking,
LeetCode,
LinkedIn,
MJ,
Permutation,
Solution
Subscribe to:
Posts (Atom)