1610. Maximum Number of Visible Points
You are given an array points
, an integer angle
, and your location
, where location = [posx, posy]
and points[i] = [xi, yi]
both denote integral coordinates on the X-Y plane.
Initially, you are facing directly east from your position. You cannot move from your position, but you can rotate. In other words, posx
and posy
cannot be changed. Your field of view in degrees is represented by angle
, determining how wide you can see from any given view direction. Let d
be the amount in degrees that you rotate counterclockwise. Then, your field of view is the inclusive range of angles [d - angle/2, d + angle/2]
.
You can see some set of points if, for each point, the angle formed by the point, your position, and the immediate east direction from your position is in your field of view.
There can be multiple points at one coordinate. There may be points at your location, and you can always see these points regardless of your rotation. Points do not obstruct your vision to other points.
Return the maximum number of points you can see.
Example 1:
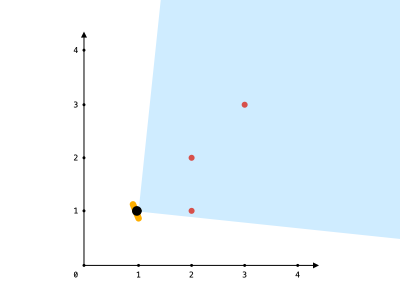
Input: points = [[2,1],[2,2],[3,3]], angle = 90, location = [1,1] Output: 3 Explanation: The shaded region represents your field of view. All points can be made visible in your field of view, including [3,3] even though [2,2] is in front and in the same line of sight.
Example 2:
Input: points = [[2,1],[2,2],[3,4],[1,1]], angle = 90, location = [1,1] Output: 4 Explanation: All points can be made visible in your field of view, including the one at your location.
Example 3:
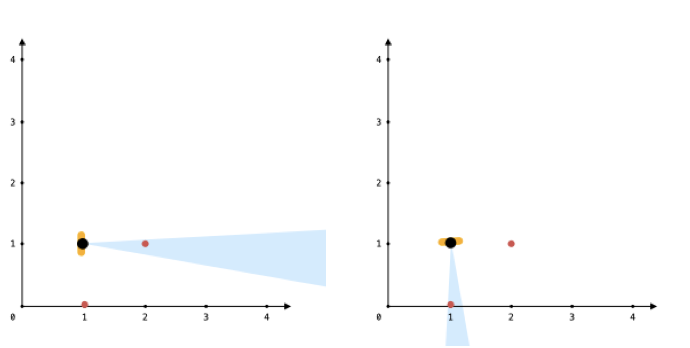
Input: points = [[1,0],[2,1]], angle = 13, location = [1,1] Output: 1 Explanation: You can only see one of the two points, as shown above.
Constraints:
1 <= points.length <= 105
points[i].length == 2
location.length == 2
0 <= angle < 360
0 <= posx, posy, xi, yi <= 100
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | class Solution { public int visiblePoints(List<List<Integer>> points, int angle, List<Integer> location) { int n = points.size(); List<Double> degress = new ArrayList<>(); int origins = 0; for(List<Integer> point : points){ int x = point.get(0) - location.get(0); int y = point.get(1) - location.get(1); double d = 0; if(x==0){ if(y==0) origins++; else if(y>0) d = 90; else d = 270; }else{ d = Math.toDegrees(Math.atan(y/(double)x)); if(x<0 && y>=0) d = 180 + d;//2 else if(x<0 && y<0) d = 180+d;//3 else if(x>0 && y<0) d = 360+d;//4 } if(x!=0 || y!=0) degress.add(d); } n = degress.size(); for(int i=n; i<2*n; ++i) degress.add(degress.get(i-n)+360); Collections.sort(degress); int l = 0, r = 0; int max = 0; while(r<2*n){ while(r<2*n && degress.get(r)-degress.get(l)<=angle) r++; max = Math.max(r-l, max); l++; } return max+origins; } } |