212. Word Search II
Hard
Given an m x n
board
of characters and a list of strings words
, return all words on the board.
Each word must be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring. The same letter cell may not be used more than once in a word.
Example 1:
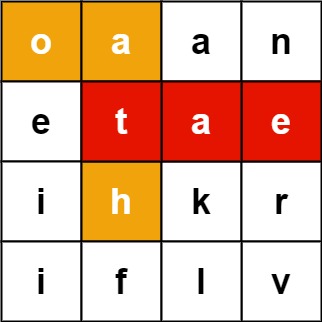
Input: board = [["o","a","a","n"],["e","t","a","e"],["i","h","k","r"],["i","f","l","v"]], words = ["oath","pea","eat","rain"] Output: ["eat","oath"]
Example 2:
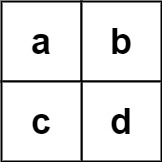
Input: board = [["a","b"],["c","d"]], words = ["abcb"] Output: []
Constraints:
m == board.length
n == board[i].length
1 <= m, n <= 12
board[i][j]
is a lowercase English letter.1 <= words.length <= 3 * 104
1 <= words[i].length <= 10
words[i]
consists of lowercase English letters.- All the strings of
words
are unique.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | class TrieNode{ public: char key; bool isTail = false; TrieNode* children[26] = {NULL}; TrieNode(){} TrieNode(char k):key(k){} }; class Trie{ public: TrieNode * root = new TrieNode(); Trie(){} void insert(string word){ TrieNode *p = root; for(char c:word){ if(p->children[c-'a']==NULL){ p->children[c-'a'] = new TrieNode(c); } p = p->children[c-'a']; } p->isTail = true; } }; class Solution { public: vector<string> findWords(vector<vector<char>>& board, vector<string>& words) { Trie trie; for(string w:words) trie.insert(w); vector<string> ret; int m = board.size(), n = board[0].size(); for(int i=0; i<m; ++i){ for(int j=0; j<n; ++j){ dfs(i, j, m, n, board, trie.root, ret, ""); } } return ret; } void dfs(int i, int j, int m, int n, vector<vector<char>>& board, TrieNode *t, vector<string> &ret, string cur){ char c = board[i][j]; board[i][j] = '#'; if(c!='#' && t->children[c-'a']!=NULL){ cur += c; if(t->children[c-'a']->isTail){ ret.push_back(cur); t->children[c-'a']->isTail = false; } if(i-1>=0) dfs(i-1, j, m, n, board, t->children[c-'a'], ret, cur); if(i+1<m) dfs(i+1, j, m, n, board, t->children[c-'a'], ret, cur); if(j-1>=0) dfs(i, j-1, m, n, board, t->children[c-'a'], ret, cur); if(j+1<n) dfs(i, j+1, m, n, board, t->children[c-'a'], ret, cur); } board[i][j] = c; } }; |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | class Solution { int[][] dirs = new int[][]{{-1,0},{1,0},{0,-1},{0,1}}; class Trie{ boolean isEnd; Trie[] children = new Trie[26]; } int m, n; Trie root; void insert(String w){ Trie p = root; for(char c : w.toCharArray()){ if(p.children[c-'a']==null){ p.children[c-'a'] = new Trie(); } p = p.children[c-'a']; } p.isEnd = true; } public List<String> findWords(char[][] board, String[] words) { m = board.length; n = board[0].length; root = new Trie(); for(String w : words) insert(w); Set<String> set = new HashSet<>(); for(int i=0; i<m; ++i){ for(int j=0; j<n; ++j){ Trie p = root; char c = board[i][j]; if(p.children[c-'a']!=null){ board[i][j] = '.'; String cur = ""+c; search(i, j, board, set, p.children[c-'a'], cur); board[i][j] = c; } } } List<String> list = new ArrayList<>(set); return list; } void search(int i, int j, char[][] board, Set<String> set, Trie p, String cur){ if(p.isEnd) set.add(cur); for(int[] d : dirs){ int ii = i+d[0], jj = j+d[1]; if(ii>=0 && ii<m && jj>=0 && jj<n && board[ii][jj]!='.'){ char c = board[ii][jj]; if(p.children[c-'a']!=null){ board[ii][jj] = '.'; search(ii, jj, board, set, p.children[c-'a'], cur+c); board[ii][jj] = c; } } } } } |
No comments:
Post a Comment