270. Closest Binary Search Tree Value
Easy
Given the root
of a binary search tree and a target
value, return the value in the BST that is closest to the target
.
Example 1:
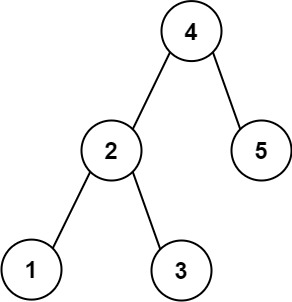
Input: root = [4,2,5,1,3], target = 3.714286 Output: 4
Example 2:
Input: root = [1], target = 4.428571 Output: 1
Constraints:
- The number of nodes in the tree is in the range
[1, 104]
. 0 <= Node.val <= 109
-109 <= target <= 109
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | /** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode() {} * TreeNode(int val) { this.val = val; } * TreeNode(int val, TreeNode left, TreeNode right) { * this.val = val; * this.left = left; * this.right = right; * } * } */ class Solution { int value = -1; public int closestValue(TreeNode root, double target) { helper(root, target); return value; } void helper(TreeNode node, double target){ if(node==null) return; if(value == -1 || Math.abs(value-target)>Math.abs(node.val-target)){ value = node.val; } if(target<node.val) helper(node.left, target); if(target>node.val) helper(node.right, target); } } |
No comments:
Post a Comment