42. Trapping Rain Water
Hard
Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it is able to trap after raining.
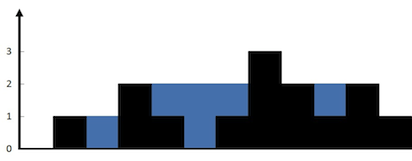
The above elevation map is represented by array [0,1,0,2,1,0,1,3,2,1,2,1]. In this case, 6 units of rain water (blue section) are being trapped. Thanks Marcos for contributing this image!
Example:
Input: [0,1,0,2,1,0,1,3,2,1,2,1] Output: 6
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | \//use tow points and compute the block+water then minus the blocks class Solution { public: int trap(vector<int>& height) { int n = height.size(), l = 0, r = n-1, level = 0, all = 0, block = 0; while(l<=r){ int new_level = min(height[l], height[r]); if(new_level>level){ all += (new_level-level)*(r-l+1); level = new_level; } if(height[l]<height[r]){ block += height[l++]; }else{ block += height[r--]; } } return all-block; } }; //use stack and count in the water every time a higher bar is found class Solution { public: int trap(vector<int>& height) { stack<int> stk; int ret = 0; for(int i=0; i<height.size(); ++i){ while(!stk.empty() && height[i]>height[stk.top()]){ int bot = height[stk.top()]; stk.pop(); if(!stk.empty()) { int top = min(height[i], height[stk.top()]); int len = i-stk.top()-1; ret += (top-bot)*len; } } stk.push(i); } return ret; } }; |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | class Solution { public int trap(int[] height) { int l = 0, r = height.length-1, level = 0;; int all = 0, block = 0; while(l<=r) { int newLevel = Math.min(height[l], height[r]); if(newLevel > level){ all += (newLevel - level) * (r-l+1); level = newLevel; } if(height[l]<height[r]) { block += height[l++]; }else{ block += height[r--]; } // System.out.println(l + " " + r " " + level + " " + newLevel + " " + all + " " + block); } return all - block; } } |
No comments:
Post a Comment