1074. Number of Submatrices That Sum to Target
Hard
Given a matrix
and a target
, return the number of non-empty submatrices that sum to target.
A submatrix x1, y1, x2, y2
is the set of all cells matrix[x][y]
with x1 <= x <= x2
and y1 <= y <= y2
.
Two submatrices (x1, y1, x2, y2)
and (x1', y1', x2', y2')
are different if they have some coordinate that is different: for example, if x1 != x1'
.
Example 1:
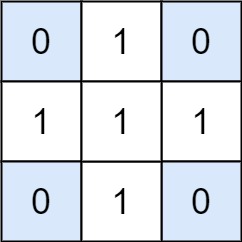
Input: matrix = [[0,1,0],[1,1,1],[0,1,0]], target = 0 Output: 4 Explanation: The four 1x1 submatrices that only contain 0.
Example 2:
Input: matrix = [[1,-1],[-1,1]], target = 0 Output: 5 Explanation: The two 1x2 submatrices, plus the two 2x1 submatrices, plus the 2x2 submatrix.
Example 3:
Input: matrix = [[904]], target = 0 Output: 0
Constraints:
1 <= matrix.length <= 100
1 <= matrix[0].length <= 100
-1000 <= matrix[i] <= 1000
-10^8 <= target <= 10^8
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | class Solution { public int numSubmatrixSumTarget(int[][] matrix, int target) { int m = matrix.length, n = matrix[0].length; int[][] A = new int[m][n]; for(int i=0; i<m; ++i){ for(int j=0; j<n; ++j){ A[i][j] = (j==0?0:A[i][j-1]) + matrix[i][j]; } } int ret = 0; for(int i=0; i<n; ++i){ for(int j=i; j<n; ++j){ Map<Integer, Integer> map = new HashMap<>();//sum, counter map.put(0, 1); int sum = 0; for(int k=0; k<m; ++k){ sum += A[k][j]-(i==0?0:A[k][i-1]); ret += map.getOrDefault(sum-target, 0); map.put(sum, map.getOrDefault(sum, 0)+1); } } } return ret; } } |
No comments:
Post a Comment